Configuration
A configuration object is required to initialize the Helpshift PC Widget similar to the Helpshift Widget. Below are the configuration options that we have.
Base Configuration
using HSWidgetLibrary
var config = new HelpshiftConfig
{
Domain = "<YOUR_DOMAIN>",
PlatformId = "<YOUR_PLATFORM_ID>",
AppId = "<YOUR_APP_ID>"
};
// Pass the configuration to the initialize API
Helpshift.Initialize(Application.streamingAssetsPath, config);
- Replace
YOUR_DOMAIN
with your Helpshift domain. For example, happyapps if your dashboard sits at happyapps.helpshift.com. Domain details can be found at Settings > SDKs (for Developers). - Replace
YOUR_PLATFORM_ID
with details found at Settings > SDKs (for Developers). - Replace
YOUR_APP_ID
with details found at Settings > Your App (eg. Helpshift Demo App) > Publish ID.
Optional Configuration
Widget Type
The WidgetType
config value controls whether both Helpcenter and Web Chat or only Helpcenter or only Web Chat should be loaded in Helpshift Widget.
The WidgetType
configuration will not work with Helpshift.Update()
API. It will work only when added with Helpshift.Initialize()
.
To show both Helpcenter and Webchat in the Helpshift Widget, use the following configuration
using HSWidgetLibrary
var config = new HelpshiftConfig
{
Domain = "<YOUR_DOMAIN>",
PlatformId = "<YOUR_PLATFORM_ID>",
AppId = "<YOUR_APP_ID>",
WidgetType = "helpcenter_and_webchat"
};
// Pass the configuration to the initialize API
Helpshift.Initialize(Application.streamingAssetsPath, config);
To show only Helpcenter in the Helpshift Widget, use the following configuration:
using HSWidgetLibrary
var config = new HelpshiftConfig
{
Domain = "<YOUR_DOMAIN>",
PlatformId = "<YOUR_PLATFORM_ID>",
AppId = "<YOUR_APP_ID>",
WidgetType = "helpcenter"
};
// Pass the configuration to the initialize API
Helpshift.Initialize(Application.streamingAssetsPath, config);
To show only Webchat in the Helpshift Widget, use the following configuration:
using HSWidgetLibrary
var config = new HelpshiftConfig
{
Domain = "<YOUR_DOMAIN>",
PlatformId = "<YOUR_PLATFORM_ID>",
AppId = "<YOUR_APP_ID>",
WidgetType = "webchat"
};
// Pass the configuration to the initialize API
Helpshift.Initialize(Application.streamingAssetsPath, config);
Theming
You can configure the Helpcenter and Web Chat's color scheme directly from the Helpshift Dashboard. For more details, refer to For Support Admins: Configure the User Experience of Helpcenter and For Support Admins: Configure the User Experience of Web Chat and in our Knowledge Base.
Bots
Bots can be enabled in Web Chat to automatically provide help and gather information from users to help your Agents resolve Issues faster. Helpshift has 3 default Bots:
- QuickSearch Bot - deflect common problems by automatically suggesting relevant FAQs to users
- Identity Bot - prompt your users for their name and/or email before they create an issue
- CSAT Bot - automatically ask for feedback after an Issue is resolved
For more details, refer to Set Up Your Web Chat Bots in our Knowledge Base.
Full Privacy
In the HelpshiftConfig
object at the time of initialization, setting the FullPrivacy
option to true
ensures COPPA compliance by:
- Disabling user-initiated screenshots (users will not be able to attach files, including images, using Web Chat).
- Making sure that Personally Identifiable Information (PII) such as name and email are not collected by Web Chat (using Identity Bot and/or the helpshiftWidgetConfig object). This means that if you set
UserName
andUserEmail
, withFullPrivacy
set totrue
, Helpshift will not use theUserName
andUserEmail
values.
Moreover, in scenarios where the user attaches objectionable content, it becomes a huge COPPA compliance concern. This option helps to solve this problem.
By default, FullPrivacy
is set to false
.
Example Embed Code
using HSWidgetLibrary
var config = new HelpshiftConfig
{
Domain = "<YOUR_DOMAIN>",
PlatformId = "<YOUR_PLATFORM_ID>",
AppId = "<YOUR_APP_ID>",
FullPrivacy = true
};
// Pass the configuration to the initialize API
Helpshift.Initialize(Application.streamingAssetsPath, config);
Initiating chat
Developers can start a new chat when the previous issue is resolved, without needing any user action like clicking the New Conversation
button or going through the post resolution flow like feedback bot for the previously resolved issue.
To do the above mentioned action, the developer will have to set InitiateChatOnLoad
config in HelpshiftWidgetConfig
and then use the Helpshift.Update() API to start the new chat when the previous issue is resolved.
Example Embed Code
using HSWidgetLibrary
var config = new HelpshiftConfig
{
Domain = "<YOUR_DOMAIN>",
PlatformId = "<YOUR_PLATFORM_ID>",
AppId = "<YOUR_APP_ID>",
InitiateChatOnLoad = true
};
// Pass the configuration to the initialize API
Helpshift.Initialize(Application.streamingAssetsPath, config);
Initial user message
Example Embed Code
using HSWidgetLibrary
var config = new HelpshiftConfig
{
Domain = "<YOUR_DOMAIN>",
PlatformId = "<YOUR_PLATFORM_ID>",
AppId = "<YOUR_APP_ID>",
InitialUserMessage = "Some initial user message"
};
// Pass the configuration to the initialize API
Helpshift.Initialize(Application.streamingAssetsPath, config);
Conversation prefill text
Example embed code:
using HSWidgetLibrary
var config = new HelpshiftConfig
{
Domain = "<YOUR_DOMAIN>",
PlatformId = "<YOUR_PLATFORM_ID>",
AppId = "<YOUR_APP_ID>",
ConversationPrefillText = "Some prefill text"
};
// Pass the configuration to the initialize API
Helpshift.Initialize(Application.streamingAssetsPath, config);
Showing a Particular FAQ Section
You can use this configuration to show FAQs from a particular FAQ section.
You will need the publish-id
of the FAQ section to use this API:
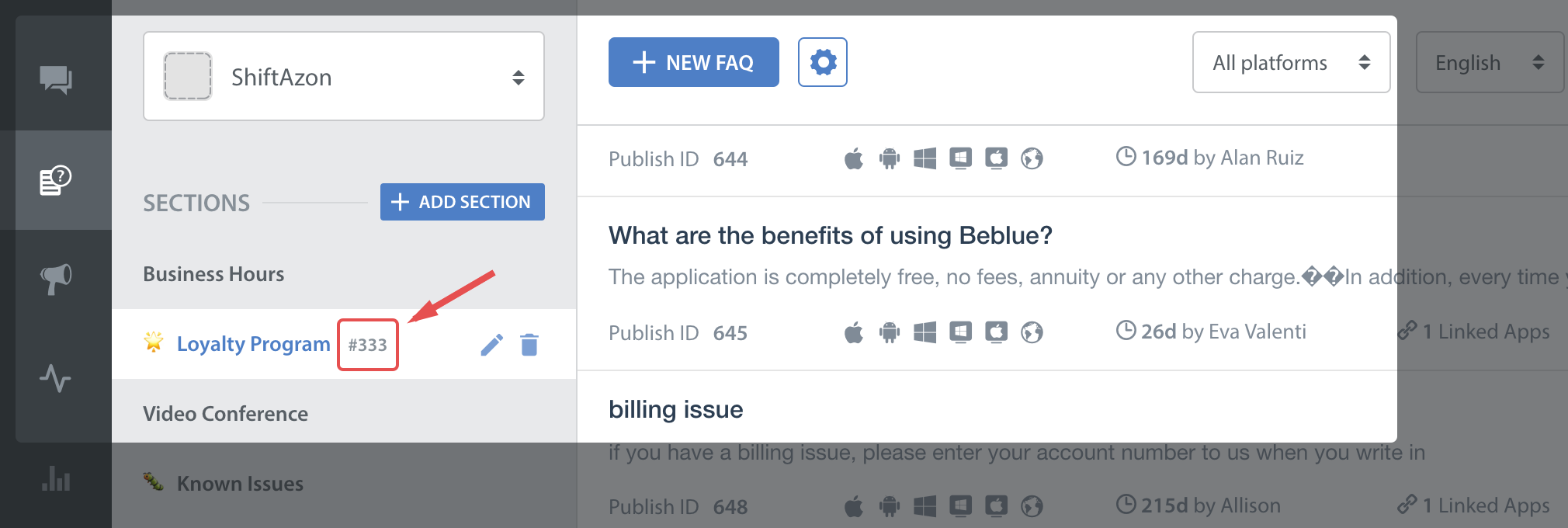
Example embed code:
using HSWidgetLibrary
var config = new HelpshiftConfig
{
SectionId = "333",
};
// Pass the configuration to the initialize API
Helpshift.Initialize(Application.streamingAssetsPath, config);
Showing a Particular FAQ
You can use this configuration to show a single FAQ.
You'll need the publish-id
of the FAQ to use this API:
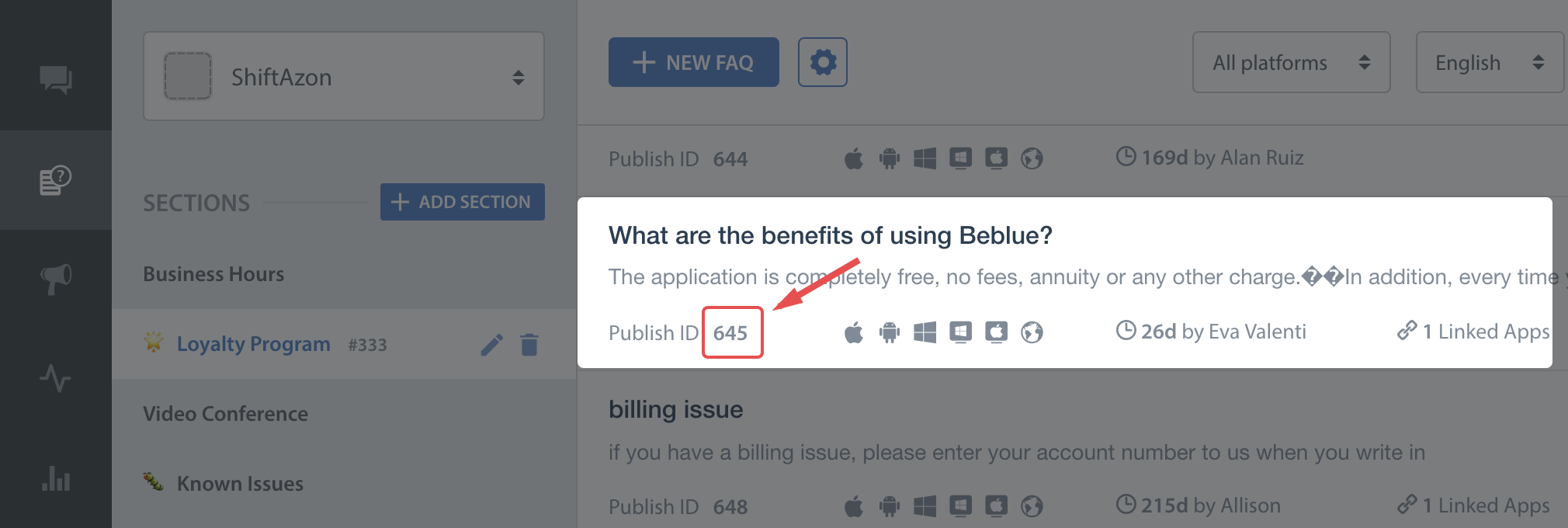
FAQ ID takes precedence over Section ID. So if both are given, FAQ will be shown.
Example embed code:
using HSWidgetLibrary
var config = new HelpshiftConfig
{
FaqId = "645",
};
// Pass the configuration to the initialize API
Helpshift.Initialize(Application.streamingAssetsPath, config);
Tags
We have FAQ filtering capability by tags. With the goal of helping the end user see focused & related content e.g. basis the user demographic or device profiles, developers can now choose this capability for FAQ filtering and showing a focused FAQ list to the right audience.
Typical cases why you would want to use FAQ filtering are :
- You want to show specific FAQs for specific audience. E.g. if you may categorize the users as ‘beginner’, ‘intermediate’ or ‘expert’ based on your business logic.
- You may want to show specific FAQs based on the device. E.g. a set of FAQs for iPad vs. iPhone.
FAQ filtering is a 2 step approach :
- FAQs need to be classified using the
<issue tags>
field on the dashboard e.g. tagsiphone
&ipad
. - Once the FAQs are tagged, they can be filtered in Helpshift Widget using the filter options described here.
Helpshift has 2 types of tags mainly ‘Issue Tags’ & ‘Search Tags’.
- Issue tags are used to filter the FAQ list in the Helpcenter on Helpshift Widget with the filter rules.
- Search tags (a.k.a Search Keywords) While searching on Helpcenter in Helpshift Widget it gives preference to these keywords. You can also use this to add alternative keywords that users might search for, but which may not exist in the FAQ title or the content.
using HSWidgetLibrary
var config = new HelpshiftConfig
{
Tags = new List<string> { "iphone", "ipad" },
};
// Pass the configuration to the initialize API
Helpshift.Initialize(Application.streamingAssetsPath, config);
Resize Modes
Determines how the Helpshift PC Widget resizes. Example values include ResizeMode.SlimRight
, ResizeMode.SlimCenter
, ResizeMode.SlimLeft
, ResizeMode.WideCenter
, and ResizeMode.FullScreen
.
ResizeMode.SlimRight
.Example embed code:
var config = new HelpshiftConfig
{
Domain = "mydomain",
PlatformId = "mydomain_platform_123",
AppId = "3",
ResizeMode = "SlimRight"
};
// Pass the configuration to the initialize API
Helpshift.Initialize(Application.streamingAssetsPath, config);
Mode Previews
Mode | Preview |
---|---|
SlimRight (Default) | ![]() |
SlimLeft | ![]() |
SlimCenter | ![]() |
WideCenter | ![]() |
FullScreen | ![]() |